<ArrayList>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
|
package com.day13;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
public class Test1 {
public static void main(String[] args) {
ArrayList<String> lists = new ArrayList<String>();
lists.add("서울");
lists.add("부산");
lists.add("대구");
Iterator<String> it = lists.iterator();
while(it.hasNext()){
System.out.print(str + " "); //가로로 찍는 방법
}
System.out.println();
System.out.println("----------------------");
ListIterator<String> it2 = lists.listIterator();
while(it2.hasNext()){
}
System.out.println();
//출력 후 데이트는 null
while(it2.hasNext()){
}
System.out.println("----------------------");
//역순으로 출력
//ListIterator<String> it3 = lists.listIterator();
while(it2.hasPrevious()){
System.out.println(it2.previous());
}
System.out.println("----------------------");
List<String> lists1 = new ArrayList<String>();
lists1.add("인천");
int n = lists1.indexOf("부산");//1
lists1.add(n+1, "광주");
for(String c : lists1){
System.out.print(c + " ");
}
System.out.println();
//------------------------------------------------
System.out.println("-----------------------");
List<String> lists2 = new ArrayList<String>();
lists2.add("자바프로그래머");
lists2.add("프레임워크");
lists2.add("스트럿츠");
lists2.add("서블릿");
lists2.add("스프링");
String str;
Iterator<String> it4 = lists2.iterator();
while(it4.hasNext()){
str = it4.next();
if(str.startsWith("서"))
System.out.println(str);
}
}
}
|
==Console==
<Map>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
|
package com.day13;
import java.util.Hashtable;
import java.util.Iterator;
//Map<키,값> 인터페이스
//Hashtable : vector와 동일(동기화를 지원)
//HashMap : ArrayList와 동일
//키는 중복값을 가질 수 없다.(키는 set이다.)
//키가 중복값을 가지면 마지막 값이 저장(수정)
//Map은 Iterator가 없다.(set의 Iterator를 빌려쓴다.)
//put(입력)
//get(출력)
//key라는 고유값을 가지고서 대상을 찾기 때문에 빠름
//반드시 key와 value값을 같이 줘야 함
public class Test2 {
public static final String tel[] = {"111-111","222-222","333-333","111-111","444-444"};//key
public static final String name[] = {"이효리","박신혜","한지민","배수지","천송이"}; //value
public static void main(String[] args) {
Hashtable<String, String> h = new Hashtable<String, String>(); //객체 생성
//map으로 변경 가능
for(int i=0;i<tel.length;i++){
h.put(tel[i], name[i]);
}
System.out.println(h);
//map의 출력 순서는 map만의 정리 방법으로 나옴(들어가는 순서가 아님)
//-----------------------------------
String str;
str = h.get("111-111"); //키를 주면 value를 반환
System.out.println(str);
//키가 존재하는지 검사
if(h.containsKey("222-222"))
System.out.println("222-222가 존재...");
else
System.out.println("222-222가 안존재...");
//value가 존재하는지 검사
if(h.containsValue("배수지"))//띄어쓰기나, 숫자, 글자가 다르면 못찾음
System.out.println("수지 여기 있어요!");
else
System.out.println("수지 없다!");
//데이터 삭제
h.remove("222-222");
if(h.contains("222-222"))
System.out.println("222-222가 존재...");
else
System.out.println("222-222가 안존재...");
//키는 Set이며, Set은 중복을 허용하지 않는 자료구조이다.
//Set은 Iterator가 존재하므로 Hashtable 또는 HashMap의
//keySet()메소드로 Iterator를 사용한다.
Iterator<String> it = h.keySet().iterator();
//Iterator<key의 자료형> 변수
while(it.hasNext()){
String value = h.get(key); //value값을 출력하는 방법
System.out.println(key + " : " + value);
}
}
}
|
==Console==
<Set>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
|
package com.day13;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Queue;
import java.util.Set;
import java.util.Stack;
public class Test3 {
public static void main(String[] args) {
//Set : 중복을 허용하지 않는다
Set<String> s = new HashSet<String>();
s.add("서울");
s.add("부산");
s.add("대구");
System.out.println(s);
Iterator<String> it = s.iterator();
while(it.hasNext()){
System.out.print(str + " ");
}
System.out.println();
//중복허용안함
s.add("서울");
System.out.println(s);
//Stack
Stack<String> st = new Stack<String>();
st.push("서울");
st.add("부산");
st.push("대구");
st.push("광주");
//출력한 데이터는 삭제됨
}
System.out.println("\n------------------");
//Queue
Queue<String> q = new LinkedList<String>(); //linkedlist : interface 구현
//interface의 일종
q.add("서울");
q.offer("부산");
q.offer("대구");
q.offer("광주");
while(q.peek()!=null){ //peek : 들어가있는 데이터가 null이 아닐때까지 꺼낼 것
System.out.print(q.poll() + " "); //poll : 데이터를 꺼내라
}
System.out.println("\n-------------------");
List<String> lists1 = new LinkedList<String>(); //interface는 다중 구현 가능
lists1.add("A");
lists1.add("B");
lists1.add("C");
lists1.add("D");
lists1.add("E");
lists1.add("F");
lists1.add("G");
lists1.add("H");
List<String> lists2 = new LinkedList<String>();
lists2.add("서울");
lists2.add("부산");
lists2.add("대구");
lists2.addAll(lists1); //list2 나오고 뒤로 list1을 붙여줌
for(String ss : lists1){
System.out.print(ss + " ");
}
System.out.println();
for(String ss : lists2){
System.out.print(ss + " ");
}
System.out.println();
System.out.println("-------------------");
//범위삭제
lists2.subList(2, 5).clear(); //index 2에서 (5-1)까지의 범위를 삭제하고 빈공간은 다시 위치 조정
for(String ss : lists2){
System.out.print(ss + " ");
}
System.out.println();
//-----------------------------------------------
String[] str = {"다","바","나","가","마","라"};
for(String ss : str)
System.out.print(ss + " ");
System.out.println();
//배열 정렬
Arrays.sort(str); //array에 s 꼭 붙여줘야 함
for(String ss : str)
System.out.print(ss + " ");
System.out.println();
}
}
/*
[List]
List<저장할 자료형> list = new ArrayList<저장할 자료형>(); //사용방법 기억
List<저장할 자료형> list = new Vector<저장할 자료형>();
ArrayList<저장할 자료형> list = new ArrayList<저장할 자료형>();
Vector<저장할 자료형> list = new Vector<저장할 자료형>();
add : 추가
size : 요소객수(데이터 갯수)
remove(index) : 삭제
clear : 전체 데이터 삭제
trimtoSize : 빈공간 삭제
Iterator<저장된자료형> it = list.Iterator();
while(it.hasNext){
저장된 자료형 value = it.next();
}
---------------------------------------------------------------------
[Map]
Map<키자료형, 저장할자료형> map = new HashMap<키자료형, 저장할자료형>(); //사용방법 기억(주로 hashmap 사용)
Map<키자료형, 저장할자료형> map = new Hashtable<키자료형, 저장할자료형>();
HashMap<키자료형, 저장할자료형> map = new HashMap<키자료형, 저장할자료형>();
Hashtable<키자료형, 저장할자료형> map = new Hashtable<키자료형, 저장할자료형>();
put(key, value) : 추가
remove(key) : 삭제
clear() : 전체삭제
Iterator<키자료형> it = map.keyset().Iterator();
while(it.hasNext){
키자료형 key = it.next();
저장된자료형 value = map.get(key);
}
*/
|
==Console==
<Generic>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
|
package com.day13;
//Generic
class Box<T>{
private T t; //정해지지 않은 자료형
public void set(T t){ //자료형에 set를 활용하여 데이터타입을 적용해줌
this.t = t;
}
public T get(){
return t;
}
}
// Generic의 단점 : 느리다
public class Test4 {
public static void main(String[] args) {
Box<Integer> b1 = new Box<Integer>();
Integer i = b1.get();
System.out.println(i);
//------------------------------------------------
Box<String> b2 = new Box<String>();
b2.set("서울");
System.out.println(s);
//-----------------------------------------------
Box b3 = new Box(); //box 안의 데이터 타입은 object
System.out.println(ii);
}
}
|
==Console==
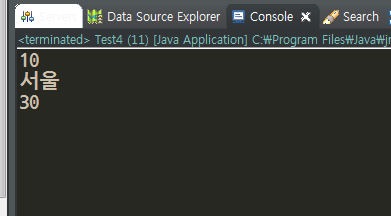
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
package com.day13;
class Box2<T>{
private T t;
public void set(T t){
this.t = t;
}
public T get(){
return t;
}
public <U> void print(U u){
System.out.println(t);
System.out.println(u);
System.out.println("t 클래스 : " + t.getClass().getName());
System.out.println("u 메소드 : " + u.getClass().getName());
}
}
public class Test5 {
public static void main(String[] args) {
Box2<Integer> b = new Box2<Integer>(); //box의 T 는 Integer가 됨
//앞의 Integer 반드시 작성 //뒤의 Integer 생략가능
b.set(new Integer(30)); //t클래스는 Integer가 됨 //T를 초기화
b.print("test"); //u클래스는 String이 됨 //메소드 초기화
b.print(50); //u클래스는 Integer가 됨
}
}
|
==Console==
<예외처리>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
|
package com.day13;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
//Exception 클래스(예외처리)
//runtime오류 : 코딩상에는 문제 없으나, 예상못한 값을 입력했을 시 발생
public class Test6 {
public static void main(String[] args) {
int a,b,result;
String oper;
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
try { //ms에서 만들어진 방법
//try부분에서 에러가 발생 시 catch에서 잡아내서 exception이 처리해서 e에 결과 출력
System.out.print("첫번째수? ");
a = Integer.parseInt(br.readLine());
System.out.print("두번째수? ");
b = Integer.parseInt(br.readLine());
System.out.print("연산자? ");
oper = br.readLine();
result = 0;
if(oper.equals("+")){
result = a + b;
}else if(oper.equals("-")){
result = a - b;
}else if(oper.equals("*")){
result = a * b;
}else if(oper.equals("/")){
result = a / b;
}
System.out.printf("%d %s %d = %d\n",a,oper,b,result);
// 예외를 나눠서 구분 가능
} catch(NumberFormatException e){
System.out.println("정수를 입력해라!!");
} catch(ArithmeticException e){
System.out.println("0으로 나누면 안돼!!");
} catch (IOException e) {
System.out.println("넌 그게 숫자로 보이냐?");
e.printStackTrace(); //에러메세지 출력
} finally{//항상 해줘야 하는 작업(코딩)을 사용
System.out.println("난 항상 보인다!!");
}
System.out.println("여기는 try 밖...");
}
}
|
==Console==
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
|
package com.day13;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Test7 {
public static String getOper() throws Exception{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String oper = null;
try{
System.out.print("연산자? ");
oper = br.readLine();
if(!oper.equals("+")&&!oper.equals("-")&&!oper.equals("*")&&!oper.equals("/")){
//throw로 예외를 의도적으로 발생 시킴
//throw를 사용하려면 반드시 throws Exception을 기술한다.
throw new Exception("연산자 입력 오류!!");
}
}catch(Exception e){
}
return oper;
}
public static void main(String[] args) {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int a,b,result;
String oper;
try {
System.out.print("첫번째수? ");
a = Integer.parseInt(br.readLine());
System.out.print("두번째수? ");
b = Integer.parseInt(br.readLine());
oper = Test7.getOper();
result = 0;
if(oper.equals("+")){
result = a + b;
}else if(oper.equals("-")){
result = a - b;
}else if(oper.equals("*")){
result = a * b;
}else if(oper.equals("/")){
result = a / b;
}
System.out.printf("%d %s %d = %d\n",a,oper,b,result);
} catch (Exception e) {
System.out.println(e.toString());
}
}
}
|
==Console==
'Java' 카테고리의 다른 글
Java Tip&Tech : 인터페이스와 추상클래스의 차이점 (0) | 2019.09.24 |
---|---|
Java Day 14 : Thread (0) | 2019.06.14 |
Java Day 12 :내부클래스,Collections Framework (0) | 2019.06.14 |
Java Day 11 : 추상 클래스, Interface (0) | 2019.06.14 |
Java Day10 :Calendar,String주요메소드,Wrapper Class,Singleton (0) | 2019.06.13 |